GitHub - orlp/pdqsort: Pattern-defeating quicksort.Pattern-defeating quicksort. Contribute to orlp/pdqsort development by creating an account on GitHub.
Visit Site
GitHub - orlp/pdqsort: Pattern-defeating quicksort.
pdqsort
Pattern-defeating quicksort (pdqsort) is a novel sorting algorithm that combines the fast average case of randomized quicksort with the fast worst case of heapsort, while achieving linear time on inputs with certain patterns. pdqsort is an extension and improvement of David Mussers introsort. All code is available for free under the zlib license.
Best Average Worst Memory Stable Deterministic
n n log n n log n log n No Yes
Usage
pdqsort
is a drop-in replacement for std::sort
.
Just replace a call to std::sort
with pdqsort
to start using pattern-defeating quicksort. If your
comparison function is branchless, you can call pdqsort_branchless
for a potential big speedup. If
you are using C++11, the type you're sorting is arithmetic and your comparison function is not given
or is std::less
/std::greater
, pdqsort
automatically delegates to pdqsort_branchless
.
Benchmark
A comparison of pdqsort and GCC's std::sort
and std::stable_sort
with various input
distributions:
Compiled with -std=c++11 -O2 -m64 -march=native
.
Visualization
A visualization of pattern-defeating quicksort sorting a ~200 element array with some duplicates. Generated using Timo Bingmann's The Sound of Sorting program, a tool that has been invaluable during the development of pdqsort. For the purposes of this visualization the cutoff point for insertion sort was lowered to 8 elements.
The best case
pdqsort is designed to run in linear time for a couple of best-case patterns. Linear time is achieved for inputs that are in strictly ascending or descending order, only contain equal elements, or are strictly in ascending order followed by one out-of-place element. There are two separate mechanisms at play to achieve this.
For equal elements a smart partitioning scheme is used that always puts equal elements in the partition containing elements greater than the pivot. When a new pivot is chosen it's compared to the greatest element in the partition before it. If they compare equal we can derive that there are no elements smaller than the chosen pivot. When this happens we switch strategy for this partition, and filter out all elements equal to the pivot.
To get linear time for the other patterns we check after every partition if any swaps were made. If no swaps were made and the partition was decently balanced we will optimistically attempt to use insertion sort. This insertion sort aborts if more than a constant amount of moves are required to sort.
The average case
On average case data where no patterns are detected pdqsort is effectively a quicksort that uses median-of-3 pivot selection, switching to insertion sort if the number of elements to be (recursively) sorted is small. The overhead associated with detecting the patterns for the best case is so small it lies within the error of measurement.
pdqsort gets a great speedup over the traditional way of implementing quicksort when sorting large arrays (1000+ elements). This is due to a new technique described in "BlockQuicksort: How Branch Mispredictions don't affect Quicksort" by Stefan Edelkamp and Armin Weiss. In short, we bypass the branch predictor by using small buffers (entirely in L1 cache) of the indices of elements that need to be swapped. We fill these buffers in a branch-free way that's quite elegant (in pseudocode):
buffer_num = 0; buffer_max_size = 64;
for (int i = 0; i < buffer_max_size; ++i) {
// With branch:
if (elements[i] < pivot) { buffer[buffer_num] = i; buffer_num++; }
// Without:
buffer[buffer_num] = i; buffer_num += (elements[i] < pivot);
}
This is only a speedup if the comparison function itself is branchless, however. By default pdqsort
will detect this if you're using C++11 or higher, the type you're sorting is arithmetic (e.g.
int
), and you're using either std::less
or std::greater
. You can explicitly request branchless
partitioning by calling pdqsort_branchless
instead of pdqsort
.
The worst case
Quicksort naturally performs bad on inputs that form patterns, due to it being a partition-based sort. Choosing a bad pivot will result in many comparisons that give little to no progress in the sorting process. If the pattern does not get broken up, this can happen many times in a row. Worse, real world data is filled with these patterns.
Traditionally the solution to this is to randomize the pivot selection of quicksort. While this technically still allows for a quadratic worst case, the chances of it happening are astronomically small. Later, in introsort, pivot selection is kept deterministic, instead switching to the guaranteed O(n log n) heapsort if the recursion depth becomes too big. In pdqsort we adopt a hybrid approach, (deterministically) shuffling some elements to break up patterns when we encounter a "bad" partition. If we encounter too many "bad" partitions we switch to heapsort.
Bad partitions
A bad partition occurs when the position of the pivot after partitioning is under 12.5% (1/8th) percentile or over 87,5% percentile - the partition is highly unbalanced. When this happens we will shuffle four elements at fixed locations for both partitions. This effectively breaks up many patterns. If we encounter more than log(n) bad partitions we will switch to heapsort.
The 1/8th percentile is not chosen arbitrarily. An upper bound of quicksorts worst case runtime can be approximated within a constant factor by the following recurrence:
T(n, p) = n + T(p(n-1), p) + T((1-p)(n-1), p)
Where n is the number of elements, and p is the percentile of the pivot after partitioning.
T(n, 1/2)
is the best case for quicksort. On modern systems heapsort is profiled to be
approximately 1.8 to 2 times as slow as quicksort. Choosing p such that T(n, 1/2) / T(n, p) ~= 1.9
as n gets big will ensure that we will only switch to heapsort if it would speed up the sorting.
p = 1/8 is a reasonably close value and is cheap to compute on every platform using a bitshift.
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here 🔥.
- 1Qt | Tools for Each Stage of Software Development Lifecycle
https://www.qt.io
All the essential Qt tools for all stages of Software Development Lifecycle: planning, design, development, testing, and deployment.
- 2Verovio
https://www.verovio.org
Music notation engraving library for MEI with MusicXML and Humdrum support and various toolkits (JavaScript, Python)
- 3Amplitude Audio SDK
https://amplitudeaudiosdk.com
A full-featured and cross-platform audio engine designed with the needs of games in mind.
- 4ISO C++ Standards Committee
https://github.com/cplusplus
ISO C++ Standards Committee has 30 repositories available. Follow their code on GitHub.
- 5Public development project of the LAMMPS MD software package
https://github.com/lammps/lammps
Public development project of the LAMMPS MD software package - GitHub - lammps/lammps: Public development project of the LAMMPS MD software package
- 6Small strings compression library
https://github.com/antirez/smaz
Small strings compression library. Contribute to antirez/smaz development by creating an account on GitHub.
- 7Fast, orthogonal, open multi-methods. Solve the Expression Problem in C++17.
https://github.com/jll63/yomm2
Fast, orthogonal, open multi-methods. Solve the Expression Problem in C++17. - jll63/yomm2
- 8simple neural network library in ANSI C
https://github.com/codeplea/genann
simple neural network library in ANSI C. Contribute to codeplea/genann development by creating an account on GitHub.
- 9Public/backup repository of the GROMACS molecular simulation toolkit. Please do not mine the metadata blindly; we use https://gitlab.com/gromacs/gromacs for code review and issue tracking.
https://github.com/gromacs/gromacs
Public/backup repository of the GROMACS molecular simulation toolkit. Please do not mine the metadata blindly; we use https://gitlab.com/gromacs/gromacs for code review and issue tracking. - gromac...
- 10A small self-contained alternative to readline and libedit that supports UTF-8 and Windows and is BSD licensed.
https://github.com/arangodb/linenoise-ng
A small self-contained alternative to readline and libedit that supports UTF-8 and Windows and is BSD licensed. - arangodb/linenoise-ng
- 11KFR | Fast, modern C++ DSP framework
https://www.kfrlib.com/
KFR | Fast, modern C++ DSP framework, DFT/FFT, Audio resampling, FIR, IIR and Biquad filters, Filter design, Tensors, Full vectorization
- 12LibU is a multiplatform utility library written in C, with APIs for handling memory allocation, networking and URI parsing, string manipulation, debugging, and logging in a very compact way, plus many other miscellaneous tasks
https://github.com/koanlogic/libu
LibU is a multiplatform utility library written in C, with APIs for handling memory allocation, networking and URI parsing, string manipulation, debugging, and logging in a very compact way, plus m...
- 13A header-only and easy to use Ini file parser for C++.
https://github.com/Rookfighter/inifile-cpp
A header-only and easy to use Ini file parser for C++. - Rookfighter/inifile-cpp
- 14libTorrent BitTorrent library
https://github.com/rakshasa/libtorrent
libTorrent BitTorrent library. Contribute to rakshasa/libtorrent development by creating an account on GitHub.
- 15SimplE Lossless Audio
https://github.com/sahaRatul/sela
SimplE Lossless Audio. Contribute to sahaRatul/sela development by creating an account on GitHub.
- 16Boost.org
https://github.com/boostorg
Boost provides free peer-reviewed portable C++ source libraries. - Boost.org
- 17The Official MongoDB driver for C language
https://github.com/mongodb/mongo-c-driver
The Official MongoDB driver for C language. Contribute to mongodb/mongo-c-driver development by creating an account on GitHub.
- 18The AI-native database built for LLM applications, providing incredibly fast hybrid search of dense vector, sparse vector, tensor (multi-vector), and full-text
https://github.com/infiniflow/infinity
The AI-native database built for LLM applications, providing incredibly fast hybrid search of dense vector, sparse vector, tensor (multi-vector), and full-text - infiniflow/infinity
- 19Simple Unit Testing for C
https://github.com/ThrowTheSwitch/Unity
Simple Unit Testing for C. Contribute to ThrowTheSwitch/Unity development by creating an account on GitHub.
- 20Basic Development Environment - a set of foundational C++ libraries used at Bloomberg.
https://github.com/bloomberg/bde
Basic Development Environment - a set of foundational C++ libraries used at Bloomberg. - bloomberg/bde
- 21C++14 evented IO libraries for high performance networking and media based applications
https://github.com/sourcey/libsourcey
C++14 evented IO libraries for high performance networking and media based applications - sourcey/libsourcey
- 22Asio C++ Library
https://github.com/chriskohlhoff/asio/
Asio C++ Library. Contribute to chriskohlhoff/asio development by creating an account on GitHub.
- 23A readline and libedit replacement that supports UTF-8, syntax highlighting, hints and Windows and is BSD licensed.
https://github.com/AmokHuginnsson/replxx
A readline and libedit replacement that supports UTF-8, syntax highlighting, hints and Windows and is BSD licensed. - AmokHuginnsson/replxx
- 24A lightweight header-only library for using Keras (TensorFlow) models in C++.
https://github.com/Dobiasd/frugally-deep
A lightweight header-only library for using Keras (TensorFlow) models in C++. - Dobiasd/frugally-deep
- 25A collection of std-like single-header C++ libraries
https://github.com/iboB/itlib
A collection of std-like single-header C++ libraries - iboB/itlib
- 26C++11 port of docopt
https://github.com/docopt/docopt.cpp
C++11 port of docopt. Contribute to docopt/docopt.cpp development by creating an account on GitHub.
- 27🎁 A glib-like multi-platform c library
https://github.com/tboox/tbox
🎁 A glib-like multi-platform c library. Contribute to tboox/tbox development by creating an account on GitHub.
- 28Audio decoding libraries for C/C++, each in a single source file.
https://github.com/mackron/dr_libs
Audio decoding libraries for C/C++, each in a single source file. - mackron/dr_libs
- 29Minimalistic MP3 decoder single header library
https://github.com/lieff/minimp3
Minimalistic MP3 decoder single header library. Contribute to lieff/minimp3 development by creating an account on GitHub.
- 30C library for cross-platform real-time audio input and output
https://github.com/andrewrk/libsoundio
C library for cross-platform real-time audio input and output - andrewrk/libsoundio
- 31header only, dependency-free deep learning framework in C++14
https://github.com/tiny-dnn/tiny-dnn
header only, dependency-free deep learning framework in C++14 - tiny-dnn/tiny-dnn
- 32Polycode is a cross-platform framework for creative code.
https://github.com/ivansafrin/Polycode
Polycode is a cross-platform framework for creative code. - ivansafrin/Polycode
- 33A simple C++ library for reading and writing audio files.
https://github.com/adamstark/AudioFile
A simple C++ library for reading and writing audio files. - adamstark/AudioFile
- 34An Open Source Implementation of the Actor Model in C++
https://github.com/actor-framework/actor-framework
An Open Source Implementation of the Actor Model in C++ - actor-framework/actor-framework
- 35Embedded Template Library
https://github.com/ETLCPP/etl
Embedded Template Library. Contribute to ETLCPP/etl development by creating an account on GitHub.
- 36analyzing petabytes of data, scientifically.
https://root.cern.ch/
An open-source data analysis framework used by high energy physics and others.
- 37Behavior Tree Starter Kit
https://github.com/aigamedev/btsk
Behavior Tree Starter Kit. Contribute to aigamedev/btsk development by creating an account on GitHub.
- 38C++ Parallel Computing and Asynchronous Networking Framework
https://github.com/sogou/workflow
C++ Parallel Computing and Asynchronous Networking Framework - sogou/workflow
- 39Open-Source Quantum Chemistry – an electronic structure package in C++ driven by Python
https://github.com/psi4/psi4
Open-Source Quantum Chemistry – an electronic structure package in C++ driven by Python - psi4/psi4
- 40The PGM-index
https://pgm.di.unipi.it
The Piecewise Geometric Model index (PGM-index) is a data structure that enables fast point and range searches in arrays of billions of items using orders of magnitude less space than traditional indexes.
- 41Hello from Velox | Velox
https://velox-lib.io/
Description will go into a meta tag in <head />
- 42A tiny boost library in C++11.
https://github.com/idealvin/coost
A tiny boost library in C++11. Contribute to idealvin/coost development by creating an account on GitHub.
- 43Minimal Rust-inspired C++20 STL replacement
https://github.com/TheNumbat/rpp
Minimal Rust-inspired C++20 STL replacement. Contribute to TheNumbat/rpp development by creating an account on GitHub.
- 44Concurrency library for C (coroutines)
https://github.com/tidwall/neco
Concurrency library for C (coroutines). Contribute to tidwall/neco development by creating an account on GitHub.
- 45Microsoft Cognitive Toolkit (CNTK), an open source deep-learning toolkit
https://github.com/Microsoft/CNTK
Microsoft Cognitive Toolkit (CNTK), an open source deep-learning toolkit - microsoft/CNTK
- 46A toolkit for making real world machine learning and data analysis applications in C++
https://github.com/davisking/dlib
A toolkit for making real world machine learning and data analysis applications in C++ - davisking/dlib
- 47A C++ static library offering a clean and simple interface to the 7-zip shared libraries.
https://github.com/rikyoz/bit7z
A C++ static library offering a clean and simple interface to the 7-zip shared libraries. - rikyoz/bit7z
- 48Kigs framework is a C++ modular multipurpose cross platform framework.
https://github.com/Kigs-framework/kigs
Kigs framework is a C++ modular multipurpose cross platform framework. - Kigs-framework/kigs
- 49Activity Indicators for Modern C++
https://github.com/p-ranav/indicators/
Activity Indicators for Modern C++. Contribute to p-ranav/indicators development by creating an account on GitHub.
- 50Open multi-methods for C++11
https://github.com/jll63/yomm11
Open multi-methods for C++11. Contribute to jll63/yomm11 development by creating an account on GitHub.
- 51A fast and flexible c++ template class for tree data structures
https://github.com/erikerlandson/st_tree
A fast and flexible c++ template class for tree data structures - erikerlandson/st_tree
- 52The most over-engineered C++ assertion library
https://github.com/jeremy-rifkin/libassert
The most over-engineered C++ assertion library. Contribute to jeremy-rifkin/libassert development by creating an account on GitHub.
- 53A powerful and cross-platform audio engine, optimized for games.
https://github.com/SparkyStudios/AmplitudeAudioSDK
A powerful and cross-platform audio engine, optimized for games. - SparkyStudios/AmplitudeAudioSDK
- 54Audio playback and capture library written in C, in a single source file.
https://github.com/mackron/miniaudio
Audio playback and capture library written in C, in a single source file. - mackron/miniaudio
- 55Boost.org asio module
https://github.com/boostorg/asio
Boost.org asio module. Contribute to boostorg/asio development by creating an account on GitHub.
- 56Free, easy, portable audio engine for games
https://github.com/jarikomppa/soloud
Free, easy, portable audio engine for games. Contribute to jarikomppa/soloud development by creating an account on GitHub.
- 57The project alpaka has moved to https://github.com/alpaka-group/alpaka
https://github.com/ComputationalRadiationPhysics/alpaka
The project alpaka has moved to https://github.com/alpaka-group/alpaka - ComputationalRadiationPhysics/alpaka
- 58BitTorrent DHT library
https://github.com/jech/dht
BitTorrent DHT library. Contribute to jech/dht development by creating an account on GitHub.
- 59An in-process SQL OLAP database management system
https://duckdb.org/
DuckDB is an in-process SQL OLAP database management system. Simple, feature-rich, fast & open source.
- 60Qt
https://github.com/qt
Official mirror of the qt-project.org Git repositories - Qt
- 61OpenCL - The Open Standard for Parallel Programming of Heterogeneous Systems
https://www.khronos.org/opencl/
OpenCL™ (Open Computing Language) is an open, royalty-free standard for cross-platform, parallel programming of diverse accelerators found in supercomputers, cloud servers, personal computers, mobile devices and embedded platforms. OpenCL greatly improves the speed and responsiveness of a wide spectrum of applications in numerous market categories including professional creative tools,
- 62Library and command line tool to detect SHA-1 collision in a file
https://github.com/cr-marcstevens/sha1collisiondetection
Library and command line tool to detect SHA-1 collision in a file - cr-marcstevens/sha1collisiondetection
- 63Concurrent data structures in C++
https://github.com/preshing/junction
Concurrent data structures in C++. Contribute to preshing/junction development by creating an account on GitHub.
- 64EASTL stands for Electronic Arts Standard Template Library. It is an extensive and robust implementation that has an emphasis on high performance.
https://github.com/electronicarts/EASTL
EASTL stands for Electronic Arts Standard Template Library. It is an extensive and robust implementation that has an emphasis on high performance. - electronicarts/EASTL
- 65【A common used C++ DAG framework】 一个通用的、无三方依赖的、跨平台的、收录于awesome-cpp的、基于流图的并行计算框架。欢迎star & fork & 交流
https://github.com/ChunelFeng/CGraph
【A common used C++ DAG framework】 一个通用的、无三方依赖的、跨平台的、收录于awesome-cpp的、基于流图的并行计算框架。欢迎star & fork & 交流 - ChunelFeng/CGraph
- 66Industry-standard navigation-mesh toolset for games
https://github.com/recastnavigation/recastnavigation
Industry-standard navigation-mesh toolset for games - recastnavigation/recastnavigation
- 67C++20 Microservice Bootstrapping Framework
https://github.com/volt-software/ichor
C++20 Microservice Bootstrapping Framework. Contribute to volt-software/Ichor development by creating an account on GitHub.
- 68LZFSE compression library and command line tool
https://github.com/lzfse/lzfse
LZFSE compression library and command line tool. Contribute to lzfse/lzfse development by creating an account on GitHub.
- 69Boost.org program_options module
https://github.com/boostorg/program_options
Boost.org program_options module. Contribute to boostorg/program_options development by creating an account on GitHub.
- 70Argument Parser for Modern C++
https://github.com/p-ranav/argparse
Argument Parser for Modern C++. Contribute to p-ranav/argparse development by creating an account on GitHub.
- 71A curses library for environments that don't fit the termcap/terminfo model.
https://github.com/wmcbrine/PDCurses
A curses library for environments that don't fit the termcap/terminfo model. - wmcbrine/PDCurses
- 72Easy and efficient audio synthesis in C++
https://github.com/TonicAudio/Tonic
Easy and efficient audio synthesis in C++. Contribute to TonicAudio/Tonic development by creating an account on GitHub.
- 73The Massively Parallel Quantum Chemistry program, MPQC, computes properties of atoms and molecules from first principles using the time independent Schrödinger equation.
https://github.com/ValeevGroup/mpqc
The Massively Parallel Quantum Chemistry program, MPQC, computes properties of atoms and molecules from first principles using the time independent Schrödinger equation. - ValeevGroup/mpqc
- 74A library for audio and music analysis, feature extraction.
https://github.com/libAudioFlux/audioFlux
A library for audio and music analysis, feature extraction. - libAudioFlux/audioFlux
- 75C++ Audio and Music DSP Library
https://github.com/micknoise/Maximilian
C++ Audio and Music DSP Library. Contribute to micknoise/Maximilian development by creating an account on GitHub.
- 76Thin, unified, C++-flavored wrappers for the CUDA APIs
https://github.com/eyalroz/cuda-api-wrappers
Thin, unified, C++-flavored wrappers for the CUDA APIs - eyalroz/cuda-api-wrappers
- 77a unified framework for modeling chemically reactive systems
https://github.com/reaktoro/reaktoro
a unified framework for modeling chemically reactive systems - reaktoro/reaktoro
- 78ImTui: Immediate Mode Text-based User Interface C++ Library
https://github.com/ggerganov/imtui
ImTui: Immediate Mode Text-based User Interface C++ Library - ggerganov/imtui
- 79Library for writing text-based user interfaces
https://github.com/nsf/termbox
Library for writing text-based user interfaces. Contribute to nsf/termbox development by creating an account on GitHub.
- 80A simple to use, composable, command line parser for C++ 11 and beyond
https://github.com/bfgroup/Lyra
A simple to use, composable, command line parser for C++ 11 and beyond - bfgroup/Lyra
- 81MariadeAnton/MiLi
https://github.com/MariadeAnton/MiLi
Contribute to MariadeAnton/MiLi development by creating an account on GitHub.
- 82Distributed machine learning platform
https://github.com/Samsung/veles
Distributed machine learning platform. Contribute to Samsung/veles development by creating an account on GitHub.
- 83The project alpaka has moved to https://github.com/alpaka-group/cupla
https://github.com/ComputationalRadiationPhysics/cupla
The project alpaka has moved to https://github.com/alpaka-group/cupla - GitHub - ComputationalRadiationPhysics/cupla: The project alpaka has moved to https://github.com/alpaka-group/cupla
- 84Open Source Document-oriented DB
https://reindexer.io/
Free NoSQL in-memory database with web interface
- 85A general-purpose lightweight C++ graph library
https://github.com/bobluppes/graaf
A general-purpose lightweight C++ graph library. Contribute to bobluppes/graaf development by creating an account on GitHub.
- 86A C++ vectorized database acceleration library aimed to optimizing query engines and data processing systems.
https://github.com/facebookincubator/velox
A C++ vectorized database acceleration library aimed to optimizing query engines and data processing systems. - facebookincubator/velox
- 87Cross-platform asynchronous I/O
https://github.com/libuv/libuv
Cross-platform asynchronous I/O. Contribute to libuv/libuv development by creating an account on GitHub.
- 88an efficient feature complete C++ bittorrent implementation
https://github.com/arvidn/libtorrent
an efficient feature complete C++ bittorrent implementation - arvidn/libtorrent
- 89A small self-contained alternative to readline and libedit
https://github.com/antirez/linenoise
A small self-contained alternative to readline and libedit - antirez/linenoise
- 90Simple and yet powerful cross-platform C library providing data structures, algorithms and much more
https://github.com/kala13x/libxutils
Simple and yet powerful cross-platform C library providing data structures, algorithms and much more - kala13x/libxutils
- 91Lightweight C++ command line option parser
https://github.com/jarro2783/cxxopts
Lightweight C++ command line option parser. Contribute to jarro2783/cxxopts development by creating an account on GitHub.
- 92uTorrent Transport Protocol library
https://github.com/bittorrent/libutp
uTorrent Transport Protocol library. Contribute to bittorrent/libutp development by creating an account on GitHub.
- 93A better and stronger spiritual successor to BZip2.
https://github.com/kspalaiologos/bzip3
A better and stronger spiritual successor to BZip2. - kspalaiologos/bzip3
- 94Abseil Common Libraries (C++)
https://github.com/abseil/abseil-cpp
Abseil Common Libraries (C++). Contribute to abseil/abseil-cpp development by creating an account on GitHub.
- 95Brotli compression format
https://github.com/google/brotli
Brotli compression format. Contribute to google/brotli development by creating an account on GitHub.
- 96NI Media is a C++ library for reading and writing audio streams.
https://github.com/NativeInstruments/ni-media
NI Media is a C++ library for reading and writing audio streams. - NativeInstruments/ni-media
- 97Multiresolution Adaptive Numerical Environment for Scientific Simulation
https://github.com/m-a-d-n-e-s-s/madness
Multiresolution Adaptive Numerical Environment for Scientific Simulation - m-a-d-n-e-s-s/madness
- 98Zstandard - Fast real-time compression algorithm
https://github.com/facebook/zstd
Zstandard - Fast real-time compression algorithm. Contribute to facebook/zstd development by creating an account on GitHub.
- 99Multi-format archive and compression library
https://github.com/libarchive/libarchive
Multi-format archive and compression library. Contribute to libarchive/libarchive development by creating an account on GitHub.
- 100Structural variant detection and association testing
https://github.com/zeeev/wham
Structural variant detection and association testing - zeeev/wham
- 101Thread-safe container for sharing data between threads
https://github.com/andreiavrammsd/cpp-channel
Thread-safe container for sharing data between threads - andreiavrammsd/cpp-channel
- 102data compression library for embedded/real-time systems
https://github.com/atomicobject/heatshrink
data compression library for embedded/real-time systems - atomicobject/heatshrink
- 103The libdispatch Project, (a.k.a. Grand Central Dispatch), for concurrency on multicore hardware
https://github.com/apple/swift-corelibs-libdispatch
The libdispatch Project, (a.k.a. Grand Central Dispatch), for concurrency on multicore hardware - apple/swift-corelibs-libdispatch
- 104Async++ concurrency framework for C++11
https://github.com/Amanieu/asyncplusplus
Async++ concurrency framework for C++11. Contribute to Amanieu/asyncplusplus development by creating an account on GitHub.
- 105THIS REPOSITORY HAS MOVED TO github.com/nvidia/cub, WHICH IS AUTOMATICALLY MIRRORED HERE.
https://github.com/NVlabs/cub
THIS REPOSITORY HAS MOVED TO github.com/nvidia/cub, WHICH IS AUTOMATICALLY MIRRORED HERE. - NVlabs/cub
- 106OpenCL based GPU accelerated SPH fluid simulation library
https://github.com/libclsph/libclsph
OpenCL based GPU accelerated SPH fluid simulation library - libclsph/libclsph
- 107High performance server-side application framework
https://github.com/scylladb/seastar
High performance server-side application framework - scylladb/seastar
- 108Fast lossless data compression in C++
https://github.com/flanglet/kanzi-cpp
Fast lossless data compression in C++. Contribute to flanglet/kanzi-cpp development by creating an account on GitHub.
- 109miniz: Single C source file zlib-replacement library, originally from code.google.com/p/miniz
https://github.com/richgel999/miniz
miniz: Single C source file zlib-replacement library, originally from code.google.com/p/miniz - richgel999/miniz
- 110A C++ GPU Computing Library for OpenCL
https://github.com/boostorg/compute
A C++ GPU Computing Library for OpenCL. Contribute to boostorg/compute development by creating an account on GitHub.
- 111CLI11 is a command line parser for C++11 and beyond that provides a rich feature set with a simple and intuitive interface.
https://github.com/CLIUtils/CLI11
CLI11 is a command line parser for C++11 and beyond that provides a rich feature set with a simple and intuitive interface. - CLIUtils/CLI11
- 112Fork of the popular zip manipulation library found in the zlib distribution.
https://github.com/zlib-ng/minizip-ng
Fork of the popular zip manipulation library found in the zlib distribution. - zlib-ng/minizip-ng
- 113A text-based widget toolkit
https://github.com/gansm/finalcut
A text-based widget toolkit. Contribute to gansm/finalcut development by creating an account on GitHub.
- 114Argh! A minimalist argument handler.
https://github.com/adishavit/argh
Argh! A minimalist argument handler. Contribute to adishavit/argh development by creating an account on GitHub.
- 115C++17 Terminal User Interface(TUI) Library.
https://github.com/a-n-t-h-o-n-y/TermOx
C++17 Terminal User Interface(TUI) Library. Contribute to a-n-t-h-o-n-y/TermOx development by creating an account on GitHub.
- 116A fast, memory efficient hash map for C++
https://github.com/greg7mdp/sparsepp
A fast, memory efficient hash map for C++. Contribute to greg7mdp/sparsepp development by creating an account on GitHub.
- 117oneAPI Deep Neural Network Library (oneDNN)
https://github.com/oneapi-src/oneDNN
oneAPI Deep Neural Network Library (oneDNN). Contribute to oneapi-src/oneDNN development by creating an account on GitHub.
- 118New generation entropy codecs : Finite State Entropy and Huff0
https://github.com/Cyan4973/FiniteStateEntropy
New generation entropy codecs : Finite State Entropy and Huff0 - Cyan4973/FiniteStateEntropy
- 119Convenient, high-performance RGB color and position control for console output
https://github.com/s9w/oof
Convenient, high-performance RGB color and position control for console output - s9w/oof
- 120Extremely Fast Compression algorithm
https://github.com/lz4/lz4
Extremely Fast Compression algorithm. Contribute to lz4/lz4 development by creating an account on GitHub.
- 121Sane C++ Libraries
https://github.com/Pagghiu/SaneCppLibraries
Sane C++ Libraries. Contribute to Pagghiu/SaneCppLibraries development by creating an account on GitHub.
- 122Header-only, event based, tiny and easy to use libuv wrapper in modern C++ - now available as also shared/static library!
https://github.com/skypjack/uvw
Header-only, event based, tiny and easy to use libuv wrapper in modern C++ - now available as also shared/static library! - skypjack/uvw
- 123Bolt is a C++ template library optimized for GPUs. Bolt provides high-performance library implementations for common algorithms such as scan, reduce, transform, and sort.
https://github.com/HSA-Libraries/Bolt
Bolt is a C++ template library optimized for GPUs. Bolt provides high-performance library implementations for common algorithms such as scan, reduce, transform, and sort. - HSA-Libraries/Bolt
- 124Framework for Enterprise Application Development in c++, HTTP1/HTTP2/HTTP3 compliant, Supports multiple server backends
https://github.com/sumeetchhetri/ffead-cpp
Framework for Enterprise Application Development in c++, HTTP1/HTTP2/HTTP3 compliant, Supports multiple server backends - GitHub - sumeetchhetri/ffead-cpp: Framework for Enterprise Application De...
- 125An Open Source Machine Learning Framework for Everyone
https://github.com/tensorflow/tensorflow
An Open Source Machine Learning Framework for Everyone - tensorflow/tensorflow
- 126The C++ Standard Library for Parallelism and Concurrency
https://github.com/STEllAR-GROUP/hpx/
The C++ Standard Library for Parallelism and Concurrency - STEllAR-GROUP/hpx
- 127ArrayFire: a general purpose GPU library.
https://github.com/arrayfire/arrayfire
ArrayFire: a general purpose GPU library. Contribute to arrayfire/arrayfire development by creating an account on GitHub.
- 128Kokkos C++ Performance Portability Programming Ecosystem: The Programming Model - Parallel Execution and Memory Abstraction
https://github.com/kokkos/kokkos
Kokkos C++ Performance Portability Programming Ecosystem: The Programming Model - Parallel Execution and Memory Abstraction - kokkos/kokkos
- 129kaldi-asr/kaldi is the official location of the Kaldi project.
https://github.com/kaldi-asr/kaldi
kaldi-asr/kaldi is the official location of the Kaldi project. - kaldi-asr/kaldi
- 130Concurrency primitives, safe memory reclamation mechanisms and non-blocking (including lock-free) data structures designed to aid in the research, design and implementation of high performance concurrent systems developed in C99+.
https://github.com/concurrencykit/ck
Concurrency primitives, safe memory reclamation mechanisms and non-blocking (including lock-free) data structures designed to aid in the research, design and implementation of high performance conc...
- 131A C++ library of Concurrent Data Structures
https://github.com/khizmax/libcds
A C++ library of Concurrent Data Structures. Contribute to khizmax/libcds development by creating an account on GitHub.
- 132Header-only C++ program options parser library
https://github.com/badaix/popl
Header-only C++ program options parser library. Contribute to badaix/popl development by creating an account on GitHub.
- 133Gzip Decompression and Random Access for Modern Multi-Core Machines
https://github.com/mxmlnkn/rapidgzip
Gzip Decompression and Random Access for Modern Multi-Core Machines - mxmlnkn/rapidgzip
- 134C++ library for writing multiplatform terminal applications
https://github.com/jupyter-xeus/cpp-terminal
C++ library for writing multiplatform terminal applications - jupyter-xeus/cpp-terminal
- 135🔥 比libevent/libuv/asio更易用的网络库。A c/c++ network library for developing TCP/UDP/SSL/HTTP/WebSocket/MQTT client/server.
https://github.com/ithewei/libhv
🔥 比libevent/libuv/asio更易用的网络库。A c/c++ network library for developing TCP/UDP/SSL/HTTP/WebSocket/MQTT client/server. - ithewei/libhv
- 136Developer-friendly Continuous Regression Testing
https://touca.io/
Touca is a continuous regression testing solution that helps software engineering teams gain confidence in their daily code changes.
- 137delta3d Open Source Engine
http://sourceforge.net/projects/delta3d/
Download delta3d Open Source Engine for free. Sorry this project is no longer being supported. This project is not currently being supported but feel free to use it as an example. delta3d is a robust simulation platform built using open standards and open source software.
- 138raftlib.io
http://raftlib.io/
Simple, easy to use stream computation library for C++.
- 139googletest/googlemock/README.md at main · google/googletest
https://github.com/google/googletest/blob/master/googlemock/README.md
GoogleTest - Google Testing and Mocking Framework. Contribute to google/googletest development by creating an account on GitHub.
- 140oneAPI DPC++ Library (oneDPL) https://software.intel.com/content/www/us/en/develop/tools/oneapi/components/dpc-library.html
https://github.com/intel/parallelstl
oneAPI DPC++ Library (oneDPL) https://software.intel.com/content/www/us/en/develop/tools/oneapi/components/dpc-library.html - GitHub - oneapi-src/oneDPL: oneAPI DPC++ Library (oneDPL) https://soft...
- 141Fork of the popular zip manipulation library found in the zlib distribution.
https://github.com/nmoinvaz/minizip
Fork of the popular zip manipulation library found in the zlib distribution. - zlib-ng/minizip-ng
- 142The fastest feature-rich C++11/14/17/20/23 single-header testing framework
https://github.com/onqtam/doctest
The fastest feature-rich C++11/14/17/20/23 single-header testing framework - doctest/doctest
- 143Lightweight, Portable, Flexible Distributed/Mobile Deep Learning with Dynamic, Mutation-aware Dataflow Dep Scheduler; for Python, R, Julia, Scala, Go, Javascript and more
https://github.com/apache/incubator-mxnet
Lightweight, Portable, Flexible Distributed/Mobile Deep Learning with Dynamic, Mutation-aware Dataflow Dep Scheduler; for Python, R, Julia, Scala, Go, Javascript and more - apache/mxnet
- 144C++React: A reactive programming library for C++11.
https://github.com/schlangster/cpp.react
C++React: A reactive programming library for C++11. - snakster/cpp.react
- 145An eventing framework for building high performance and high scalability systems in C.
https://github.com/facebook/libphenom
An eventing framework for building high performance and high scalability systems in C. - facebookarchive/libphenom
- 146Facebook AI Research's Automatic Speech Recognition Toolkit
https://github.com/facebookresearch/wav2letter/
Facebook AI Research's Automatic Speech Recognition Toolkit - GitHub - flashlight/wav2letter: Facebook AI Research's Automatic Speech Recognition Toolkit
- 147C++ library and cmdline tools for parsing and manipulating VCF files with python and zig bindings
https://github.com/ekg/vcflib
C++ library and cmdline tools for parsing and manipulating VCF files with python and zig bindings - vcflib/vcflib
- 148🎵 Music notation engraving library for MEI with MusicXML and Humdrum support and various toolkits (JavaScript, Python)
https://github.com/rism-ch/verovio
🎵 Music notation engraving library for MEI with MusicXML and Humdrum support and various toolkits (JavaScript, Python) - rism-digital/verovio
- 149A C library for reading and writing sound files containing sampled audio data.
https://github.com/erikd/libsndfile/
A C library for reading and writing sound files containing sampled audio data. - libsndfile/libsndfile
- 150JUCE is an open-source cross-platform C++ application framework for desktop and mobile applications, including VST, VST3, AU, AUv3, LV2 and AAX audio plug-ins.
https://github.com/julianstorer/JUCE
JUCE is an open-source cross-platform C++ application framework for desktop and mobile applications, including VST, VST3, AU, AUv3, LV2 and AAX audio plug-ins. - juce-framework/JUCE
- 151Chaste
http://www.cs.ox.ac.uk/chaste/
Chaste
- 152raylib
http://www.raylib.com/
raylib is a simple and easy-to-use library to enjoy videogames programming.
- 153Open Ecosystem
https://01.org/onednn
Access technologies from partnerships with the community and leaders. Everything open source at Intel. We have a lot to share and a lot to learn.
- 154oneAPI Threading Building Blocks (oneTBB)
https://www.threadingbuildingblocks.org/
oneAPI Threading Building Blocks (oneTBB). Contribute to oneapi-src/oneTBB development by creating an account on GitHub.
- 155Home - OpenMP
http://openmp.org/
yes
- 156TileDB -The Modern Database
https://tiledb.io/
TileDB is the modern data stack in a box. All data, code, and compute in a single product.
- 157PLATINUMTOTO | SITUS JUDI SLOT GACOR SERVER RUSIA AUTO MAXWIN!!!
http://cmldev.net/
PLATINUMTOTO adalah situs judi slot gacor maxwin server RUSIA, yang memberikan pengalaman berjudi slot gacor gampang menang auto maxwin!!!
- 158A fast image processing library with low memory needs.
http://www.vips.ecs.soton.ac.uk/
A fast image processing library with low memory needs. - libvips/libvips
- 159Home
http://opencv.org/
OpenCV provides a real-time optimized Computer Vision library, tools, and hardware. It also supports model execution for Machine Learning (ML) and Artificial Intelligence (AI).
- 160libjson
http://sourceforge.net/projects/libjson/
Download libjson for free. A JSON reader and writer which is super-effiecient and usually runs circles around other JSON libraries. It's highly customizable to optimize for your particular project, and very lightweight.
- 161The GTK Project - A free and open-source cross-platform widget toolkit
http://www.gtk.org/
GTK is a free and open-source cross-platform widget toolkit for creating graphical user interfaces.
- 162Magnum Engine
http://magnum.graphics
Lightweight and modular C++11/C++14 graphics middleware for games and data visualization
- 163Panda3D | Open Source Framework for 3D Rendering & Games
http://www.panda3d.org/
Panda3D is an open-source, cross-platform, completely free-to-use engine for realtime 3D games, visualizations, simulations, experiments — you name it! Its rich feature set readily tailors to your specific workflow and development needs.
- 164ICU - International Components for Unicode
http://site.icu-project.org/
News 2024-04-17: ICU 75 is now available. It updates to CLDR 45 (beta blog) locale data with new locales and various additions and corrections. C++ code now requires C++17 and is being made more robust. The CLDR MessageFormat 2.0 specification is now in technology preview, together with a
- 165gRPC
http://www.grpc.io/
A high-performance, open source universal RPC framework
- 166CrowCpp
https://crowcpp.org
A Fast and Easy to use microframework for the web.
- 167Meeting Cpp
https://www.youtube.com/user/MeetingCPP/videos
Meeting C++ is an independent platform for C++, supporting the C++ community by sharing news, blogs and events for C++. Details on the yearly Meeting C++ Conference can be found on the website https://meetingcpp.com
- 168A sort wrapper enabling both use of random-access sorting on non-random access containers, and increased performance for the sorting of large types.
https://github.com/mattreecebentley/plf_indiesort
A sort wrapper enabling both use of random-access sorting on non-random access containers, and increased performance for the sorting of large types. - mattreecebentley/plf_indiesort
- 169Open Watcom V2
https://github.com/open-watcom
Open Watcom V2 has 6 repositories available. Follow their code on GitHub.
- 170CppCon
https://www.youtube.com/user/CppCon/videos
Visit cppcon.org for details on next year's conference. CppCon sponsors have made it possible to record and freely distribute over 1000 sessions from the first CppCon in 2014 to the present. We hope you enjoy them!
- 171Protocol Buffers implementation in C
https://github.com/protobuf-c/protobuf-c
Protocol Buffers implementation in C. Contribute to protobuf-c/protobuf-c development by creating an account on GitHub.
- 172C++ GUI with Qt Playlist
https://www.youtube.com/playlist?list=PLD0D54219E5F2544D
Official Playlist for thenewboston C++ GUI with Qt tutorials!
- 173ZXing ("Zebra Crossing") barcode scanning library for Java, Android
https://github.com/zxing/zxing/
ZXing ("Zebra Crossing") barcode scanning library for Java, Android - zxing/zxing
- 174A YAML parser and emitter in C++
https://github.com/jbeder/yaml-cpp
A YAML parser and emitter in C++. Contribute to jbeder/yaml-cpp development by creating an account on GitHub.
- 175"interesting" VM in C. Let's see how this goes.
https://github.com/tekknolagi/carp
"interesting" VM in C. Let's see how this goes. Contribute to tekknolagi/carp development by creating an account on GitHub.
- 176Boost.org serialization module
https://github.com/boostorg/serialization
Boost.org serialization module. Contribute to boostorg/serialization development by creating an account on GitHub.
- 177Parsing Expression Grammar Template Library
https://github.com/taocpp/PEGTL
Parsing Expression Grammar Template Library. Contribute to taocpp/PEGTL development by creating an account on GitHub.
- 178A C++ Discord API Library for Bots - D++ - The lightweight C++ Discord API Library
https://dpp.dev
A lightweight C++ Discord API library supporting the entire Discord API, including Slash Commands, Voice/Audio, Sharding, Clustering and more!
- 179Full C++17 course
https://www.youtube.com/playlist?list=PLwhKb0RIaIS1sJkejUmWj-0lk7v_xgCuT
This playlist covers what one needs to know about coding in C++ 17. We start with a program that prints the "hello world" to screen and progressively dive in...
- 180Asio C++ Library
https://github.com/chriskohlhoff/asio/
Asio C++ Library. Contribute to chriskohlhoff/asio development by creating an account on GitHub.
- 181Single header YAML 1.0 C++11 serializer/deserializer.
https://github.com/jimmiebergmann/mini-yaml
Single header YAML 1.0 C++11 serializer/deserializer. - jimmiebergmann/mini-yaml
- 182C++ Programming Tutorials Playlist
https://www.youtube.com/playlist?list=PLAE85DE8440AA6B83
thenewboston Official Buckys C++ Programming Tutorials Playlist!
- 183Slides and other materials from CppCon 2020
https://github.com/CppCon/CppCon2020
Slides and other materials from CppCon 2020. Contribute to CppCon/CppCon2020 development by creating an account on GitHub.
- 184Eric Niebler
http://ericniebler.com/
Judge me by my C++, not my WordPress
- 185C++ Library Manager for Windows, Linux, and MacOS
https://github.com/microsoft/vcpkg
C++ Library Manager for Windows, Linux, and MacOS. Contribute to microsoft/vcpkg development by creating an account on GitHub.
- 186C++ Programming Tutorials from thenewboston
https://www.youtube.com/playlist?list=PLF541C2C1F671AEF6
These are all of my C++ programming tutorials
- 187libsigc++ implements a typesafe callback system for standard C++. It allows you to define signals and to connect those signals to any callback function, either global or a member function, regardless of whether it is static or virtual.
https://github.com/libsigcplusplus/libsigcplusplus
libsigc++ implements a typesafe callback system for standard C++. It allows you to define signals and to connect those signals to any callback function, either global or a member function, regardle...
- 188Cross-platform, Serial Port library written in C++
https://github.com/wjwwood/serial
Cross-platform, Serial Port library written in C++ - wjwwood/serial
- 189C Programming Tutorials
https://www.youtube.com/playlist?list=PL78280D6BE6F05D34
All if my C programming tutorials are right here!
- 190Bo Qian
https://www.youtube.com/user/BoQianTheProgrammer/playlists
The purpose of this channel is to teach various C++ programming topics in a short video format. You are welcomed to provide feedbacks so I can constantly improve the videos.
- 191a small C library for x86 CPU detection and feature extraction
https://github.com/anrieff/libcpuid
a small C library for x86 CPU detection and feature extraction - anrieff/libcpuid
- 192Awesome C Programming Tutorials in Hi Def [HD]
https://www.youtube.com/playlist?list=PLCB9F975ECF01953C
This is a collection of detailed C Programming Language Tutorials for Beginners and New Programmers. If you go through these tutorials you should have a fair...
- 193Catalog of C++ conferences worldwide
https://github.com/eoan-ermine/cpp-conferences
Catalog of C++ conferences worldwide. Contribute to eoan-ermine/cpp-conferences development by creating an account on GitHub.
- 194C++ package retrieval
https://github.com/pfultz2/cget
C++ package retrieval. Contribute to pfultz2/cget development by creating an account on GitHub.
- 195Slides and other materials from CppCon 2017
https://github.com/CppCon/CppCon2017
Slides and other materials from CppCon 2017. Contribute to CppCon/CppCon2017 development by creating an account on GitHub.
- 196A C++14 cheat-sheet on lvalues, rvalues, xvalues, and more
https://github.com/jeaye/value-category-cheatsheet
A C++14 cheat-sheet on lvalues, rvalues, xvalues, and more - jeaye/value-category-cheatsheet
- 197C++ Faker library for generating fake (but realistic) data.
https://github.com/cieslarmichal/faker-cxx
C++ Faker library for generating fake (but realistic) data. - cieslarmichal/faker-cxx
- 198NIH Utility Library
https://github.com/keybuk/libnih
NIH Utility Library. Contribute to keybuk/libnih development by creating an account on GitHub.
- 199Yet Another Serialization
https://github.com/niXman/yas
Yet Another Serialization. Contribute to niXman/yas development by creating an account on GitHub.
- 200A C++11 library for serialization
https://github.com/USCiLab/cereal
A C++11 library for serialization. Contribute to USCiLab/cereal development by creating an account on GitHub.
- 201A C++ header-only parser for the PLY file format. Parse .ply happily!
https://github.com/nmwsharp/happly
A C++ header-only parser for the PLY file format. Parse .ply happily! - nmwsharp/happly
- 202TinyVM is a small, fast, lightweight virtual machine written in pure ANSI C.
https://github.com/jakogut/tinyvm
TinyVM is a small, fast, lightweight virtual machine written in pure ANSI C. - jakogut/tinyvm
- 203TinyXML2 is a simple, small, efficient, C++ XML parser that can be easily integrated into other programs.
https://github.com/leethomason/tinyxml2
TinyXML2 is a simple, small, efficient, C++ XML parser that can be easily integrated into other programs. - leethomason/tinyxml2
- 204A safe and fast high-level and low-level character input/output library for bare-metal and RTOS based embedded systems with a very small binary footprint.
https://github.com/Viatorus/emio
A safe and fast high-level and low-level character input/output library for bare-metal and RTOS based embedded systems with a very small binary footprint. - Viatorus/emio
- 205Open Source H.264 Codec
https://github.com/cisco/openh264
Open Source H.264 Codec . Contribute to cisco/openh264 development by creating an account on GitHub.
- 206A header-only library for C++(0x) that allows automagic pretty-printing of any container.
https://github.com/louisdx/cxx-prettyprint
A header-only library for C++(0x) that allows automagic pretty-printing of any container. - louisdx/cxx-prettyprint
- 207gcc-poison
https://github.com/leafsr/gcc-poison
gcc-poison. Contribute to leafsr/gcc-poison development by creating an account on GitHub.
- 208Boost.org asio module
https://github.com/boostorg/asio
Boost.org asio module. Contribute to boostorg/asio development by creating an account on GitHub.
- 209Bond is a cross-platform framework for working with schematized data. It supports cross-language de/serialization and powerful generic mechanisms for efficiently manipulating data. Bond is broadly used at Microsoft in high scale services.
https://github.com/Microsoft/bond
Bond is a cross-platform framework for working with schematized data. It supports cross-language de/serialization and powerful generic mechanisms for efficiently manipulating data. Bond is broadly ...
- 210A fast, portable, simple, and free C/C++ IDE
https://github.com/Embarcadero/Dev-Cpp
A fast, portable, simple, and free C/C++ IDE. Contribute to Embarcadero/Dev-Cpp development by creating an account on GitHub.
- 211A C++ Web Framework built on top of Qt, using the simple approach of Catalyst (Perl) framework.
https://github.com/cutelyst/cutelyst
A C++ Web Framework built on top of Qt, using the simple approach of Catalyst (Perl) framework. - cutelyst/cutelyst
- 212a small protobuf implementation in C
https://github.com/protocolbuffers/upb
a small protobuf implementation in C. Contribute to protocolbuffers/upb development by creating an account on GitHub.
- 213The Evil License Manager
https://github.com/avati/libevil
The Evil License Manager. Contribute to avati/libevil development by creating an account on GitHub.
- 214Cista is a simple, high-performance, zero-copy C++ serialization & reflection library.
https://github.com/felixguendling/cista
Cista is a simple, high-performance, zero-copy C++ serialization & reflection library. - felixguendling/cista
- 215Your binary serialization library
https://github.com/fraillt/bitsery
Your binary serialization library. Contribute to fraillt/bitsery development by creating an account on GitHub.
- 216Boost.org property_tree module
https://github.com/boostorg/property_tree
Boost.org property_tree module. Contribute to boostorg/property_tree development by creating an account on GitHub.
- 217Automatically exported from code.google.com/p/vartypes
https://github.com/szi/vartypes
Automatically exported from code.google.com/p/vartypes - szi/vartypes
- 218VerbalExpressions/QtVerbalExpressions
https://github.com/VerbalExpressions/QtVerbalExpressions
Contribute to VerbalExpressions/QtVerbalExpressions development by creating an account on GitHub.
- 219collection of C/C++ programs that try to get compilers to exploit undefined behavior
https://github.com/regehr/ub-canaries
collection of C/C++ programs that try to get compilers to exploit undefined behavior - regehr/ub-canaries
- 220MessagePack implementation for C and C++ / msgpack.org[C/C++]
https://github.com/msgpack/msgpack-c
MessagePack implementation for C and C++ / msgpack.org[C/C++] - msgpack/msgpack-c
- 221:fish_cake: A new take on polymorphism
https://github.com/iboB/dynamix
:fish_cake: A new take on polymorphism. Contribute to iboB/dynamix development by creating an account on GitHub.
- 222Cap'n Proto serialization/RPC system - core tools and C++ library
https://github.com/capnproto/capnproto
Cap'n Proto serialization/RPC system - core tools and C++ library - capnproto/capnproto
- 223A C/C++ header to help move #ifdefs out of your code
https://github.com/nemequ/hedley
A C/C++ header to help move #ifdefs out of your code - nemequ/hedley
- 224A Small C Compiler
https://github.com/rui314/8cc
A Small C Compiler. Contribute to rui314/8cc development by creating an account on GitHub.
- 225Apache Xalan C
https://github.com/apache/xalan-c
Apache Xalan C. Contribute to apache/xalan-c development by creating an account on GitHub.
- 226C++ Cheat Sheets & Infographics
https://hackingcpp.com/cpp/cheat_sheets.html
Graphics and cheat sheets, each capturing one aspect of C++: algorithms/containers/STL, language basics, libraries, best practices, terminology (信息图表和备忘录).
- 227A client/server indexer for c/c++/objc[++] with integration for Emacs based on clang.
https://github.com/Andersbakken/rtags
A client/server indexer for c/c++/objc[++] with integration for Emacs based on clang. - Andersbakken/rtags
- 228Pattern-defeating quicksort.
https://github.com/orlp/pdqsort
Pattern-defeating quicksort. Contribute to orlp/pdqsort development by creating an account on GitHub.
- 229Formatted C++20 stdlib man pages (cppreference)
https://github.com/jeaye/stdman
Formatted C++20 stdlib man pages (cppreference). Contribute to jeaye/stdman development by creating an account on GitHub.
- 230Online editor and compiler
https://paiza.io/en
Paiza.IO is online editor and compiler. Java, Ruby, Python, PHP, Perl, Swift, JavaScript... You can use for learning programming, scraping web sites, or writing batch
- 231Xcode - Apple Developer
https://developer.apple.com/xcode/
Xcode includes everything you need to develop, test, and distribute apps across all Apple platforms.
- 232Vireo is a lightweight and versatile video processing library written in C++11
https://github.com/twitter/vireo/
Vireo is a lightweight and versatile video processing library written in C++11 - twitter/vireo
- 233Easy to use and fast C++ CRC library.
https://github.com/d-bahr/CRCpp
Easy to use and fast C++ CRC library. Contribute to d-bahr/CRCpp development by creating an account on GitHub.
- 234CCTZ is a C++ library for translating between absolute and civil times using the rules of a time zone.
https://github.com/google/cctz
CCTZ is a C++ library for translating between absolute and civil times using the rules of a time zone. - google/cctz
- 235Rapid fuzzy string matching in C++ using the Levenshtein Distance
https://github.com/rapidfuzz/rapidfuzz-cpp
Rapid fuzzy string matching in C++ using the Levenshtein Distance - rapidfuzz/rapidfuzz-cpp
- 236Boost.org signals2 module
https://github.com/boostorg/signals2
Boost.org signals2 module. Contribute to boostorg/signals2 development by creating an account on GitHub.
- 237Mobile robot simulator
https://github.com/rtv/Stage
Mobile robot simulator. Contribute to rtv/Stage development by creating an account on GitHub.
- 238Visual Studio Code - Code Editing. Redefined
https://code.visualstudio.com
Visual Studio Code is a code editor redefined and optimized for building and debugging modern web and cloud applications. Visual Studio Code is free and available on your favorite platform - Linux, macOS, and Windows.
- 239scanf for modern C++
https://github.com/eliaskosunen/scnlib
scanf for modern C++. Contribute to eliaskosunen/scnlib development by creating an account on GitHub.
- 240A date and time library based on the C++11/14/17 <chrono> header
https://github.com/HowardHinnant/date
A date and time library based on the C++11/14/17 <chrono> header - HowardHinnant/date
- 241universal serialization engine
https://github.com/qicosmos/iguana
universal serialization engine. Contribute to qicosmos/iguana development by creating an account on GitHub.
- 242Sorting algorithms & related tools for C++14
https://github.com/Morwenn/cpp-sort
Sorting algorithms & related tools for C++14. Contribute to Morwenn/cpp-sort development by creating an account on GitHub.
- 243The C++ REST SDK is a Microsoft project for cloud-based client-server communication in native code using a modern asynchronous C++ API design. This project aims to help C++ developers connect to and interact with services.
https://github.com/Microsoft/cpprestsdk
The C++ REST SDK is a Microsoft project for cloud-based client-server communication in native code using a modern asynchronous C++ API design. This project aims to help C++ developers connect to an...
- 244Compile and execute C "scripts" in one go!
https://github.com/ryanmjacobs/c
Compile and execute C "scripts" in one go! Contribute to ryanmjacobs/c development by creating an account on GitHub.
- 245TreeFrog Framework : High-speed C++ MVC Framework for Web Application
https://github.com/treefrogframework/treefrog-framework
TreeFrog Framework : High-speed C++ MVC Framework for Web Application - treefrogframework/treefrog-framework
- 246A simple C++ header-only template library implementing matching using wildcards
https://github.com/zemasoft/wildcards/
A simple C++ header-only template library implementing matching using wildcards - zemasoft/wildcards
- 247A modern formatting library
https://github.com/fmtlib/fmt
A modern formatting library. Contribute to fmtlib/fmt development by creating an account on GitHub.
- 248An open-source SDK for PSP homebrew development.
https://github.com/pspdev/pspsdk
An open-source SDK for PSP homebrew development. Contribute to pspdev/pspsdk development by creating an account on GitHub.
- 249Protocol Buffers with small code size
https://github.com/nanopb/nanopb
Protocol Buffers with small code size. Contribute to nanopb/nanopb development by creating an account on GitHub.
- 250A C/C++ minor mode for Emacs powered by libclang
https://github.com/Sarcasm/irony-mode
A C/C++ minor mode for Emacs powered by libclang. Contribute to Sarcasm/irony-mode development by creating an account on GitHub.
- 251Serial Port Programming in C++
https://github.com/crayzeewulf/libserial
Serial Port Programming in C++. Contribute to crayzeewulf/libserial development by creating an account on GitHub.
- 252Production-ready C++ Asynchronous Framework with rich functionality
https://github.com/userver-framework/userver
Production-ready C++ Asynchronous Framework with rich functionality - userver-framework/userver
- 253A Fast and Easy to use microframework for the web.
https://github.com/CrowCpp/Crow
A Fast and Easy to use microframework for the web. - CrowCpp/Crow
- 254A standalone and lightweight C library
https://github.com/attractivechaos/klib
A standalone and lightweight C library. Contribute to attractivechaos/klib development by creating an account on GitHub.
- 255Functional programming style pattern-matching library for C++
https://github.com/solodon4/Mach7
Functional programming style pattern-matching library for C++ - solodon4/Mach7
- 256Embedded C/C++ web server
https://github.com/civetweb/civetweb
Embedded C/C++ web server. Contribute to civetweb/civetweb development by creating an account on GitHub.
- 257Telegram Bot C++ Library
https://github.com/baderouaich/tgbotxx
Telegram Bot C++ Library. Contribute to baderouaich/tgbotxx development by creating an account on GitHub.
- 258Your high performance web application C framework
https://github.com/boazsegev/facil.io
Your high performance web application C framework. Contribute to boazsegev/facil.io development by creating an account on GitHub.
- 259🌱Light and powerful C++ web framework for highly scalable and resource-efficient web application. It's zero-dependency and easy-portable.
https://github.com/oatpp/oatpp
🌱Light and powerful C++ web framework for highly scalable and resource-efficient web application. It's zero-dependency and easy-portable. - oatpp/oatpp
- 260Visual Studio Code
https://github.com/microsoft/vscode
Visual Studio Code. Contribute to microsoft/vscode development by creating an account on GitHub.
- 261The password hash Argon2, winner of PHC
https://github.com/P-H-C/phc-winner-argon2
The password hash Argon2, winner of PHC . Contribute to P-H-C/phc-winner-argon2 development by creating an account on GitHub.
- 262Header-only C++11 library to encode/decode base64, base64url, base32, base32hex and hex (a.k.a. base16) as specified in RFC 4648, plus Crockford's base32. MIT licensed with consistent, flexible API.
https://github.com/tplgy/cppcodec
Header-only C++11 library to encode/decode base64, base64url, base32, base32hex and hex (a.k.a. base16) as specified in RFC 4648, plus Crockford's base32. MIT licensed with consistent, flexible...
- 263Pretty Printer for Modern C++
https://github.com/p-ranav/pprint
Pretty Printer for Modern C++. Contribute to p-ranav/pprint development by creating an account on GitHub.
- 264Tiny XML library.
https://github.com/michaelrsweet/mxml
Tiny XML library. Contribute to michaelrsweet/mxml development by creating an account on GitHub.
- 265stb single-file public domain libraries for C/C++
https://github.com/nothings/stb
stb single-file public domain libraries for C/C++. Contribute to nothings/stb development by creating an account on GitHub.
- 266A Template Engine for Modern C++
https://github.com/pantor/inja
A Template Engine for Modern C++. Contribute to pantor/inja development by creating an account on GitHub.
- 267A cross-platform Qt IDE
https://github.com/qt-creator/qt-creator
A cross-platform Qt IDE. Contribute to qt-creator/qt-creator development by creating an account on GitHub.
- 268CSerialPort - lightweight cross-platform serial port library for C++/C/C#/Java/Python/Node.js/Electron
https://github.com/itas109/CSerialPort
CSerialPort - lightweight cross-platform serial port library for C++/C/C#/Java/Python/Node.js/Electron - GitHub - itas109/CSerialPort: CSerialPort - lightweight cross-platform serial port library ...
- 269:zap: The Mobile Robot Programming Toolkit (MRPT)
https://github.com/mrpt/mrpt/
:zap: The Mobile Robot Programming Toolkit (MRPT). Contribute to MRPT/mrpt development by creating an account on GitHub.
- 270Open h.265 video codec implementation.
https://github.com/strukturag/libde265
Open h.265 video codec implementation. Contribute to strukturag/libde265 development by creating an account on GitHub.
- 271🦘 A dependency injection container for C++11, C++14 and later
https://github.com/gracicot/kangaru
🦘 A dependency injection container for C++11, C++14 and later - gracicot/kangaru
- 272Bear is a tool that generates a compilation database for clang tooling.
https://github.com/rizsotto/Bear
Bear is a tool that generates a compilation database for clang tooling. - rizsotto/Bear
- 273ita1024 / waf · GitLab
https://gitlab.com/ita1024/waf
The Waf build system
- 274MicroPython - a lean and efficient Python implementation for microcontrollers and constrained systems
https://github.com/micropython/micropython
MicroPython - a lean and efficient Python implementation for microcontrollers and constrained systems - micropython/micropython
- 275Protocol Buffers - Google's data interchange format
https://github.com/protocolbuffers/protobuf
Protocol Buffers - Google's data interchange format - protocolbuffers/protobuf
- 276C++ Discord API Bot Library - D++ is Lightweight and scalable for small and huge bots!
https://github.com/brainboxdotcc/DPP
C++ Discord API Bot Library - D++ is Lightweight and scalable for small and huge bots! - brainboxdotcc/DPP
- 277cppit / jucipp · GitLab
https://gitlab.com/cppit/jucipp
juCi++: a lightweight, cross-platform IDE
- 278A header only library for creating and validating json web tokens in c++
https://github.com/Thalhammer/jwt-cpp
A header only library for creating and validating json web tokens in c++ - Thalhammer/jwt-cpp
- 279FlatBuffers: Memory Efficient Serialization Library
https://github.com/google/flatbuffers
FlatBuffers: Memory Efficient Serialization Library - google/flatbuffers
- 280Semantic version in ANSI C
https://github.com/h2non/semver.c
Semantic version in ANSI C. Contribute to h2non/semver.c development by creating an account on GitHub.
- 281awesome-cpp/books.md at master · fffaraz/awesome-cpp
https://github.com/fffaraz/awesome-cpp/blob/master/books.md
A curated list of awesome C++ (or C) frameworks, libraries, resources, and shiny things. Inspired by awesome-... stuff. - fffaraz/awesome-cpp
- 282awesome-cpp/videos.md at master · fffaraz/awesome-cpp
https://github.com/fffaraz/awesome-cpp/blob/master/videos.md
A curated list of awesome C++ (or C) frameworks, libraries, resources, and shiny things. Inspired by awesome-... stuff. - fffaraz/awesome-cpp
- 283CppDepend - Boost Your C and C++ Code Quality.
https://www.cppdepend.com/
Improve your C and C++ code quality with CppDepend, the leading static analysis and code quality tool. Try it now and experience cleaner, more maintainable code!
- 284Jinja2 C++ (and for C++) almost full-conformance template engine implementation
https://github.com/jinja2cpp/Jinja2Cpp
Jinja2 C++ (and for C++) almost full-conformance template engine implementation - jinja2cpp/Jinja2Cpp
- 285Quick C++ Benchmarks
https://quick-bench.com/
Quickly benchmark C++ runtimes
- 286A modern C++ library for type-safe environment variable parsing
https://github.com/ph3at/libenvpp
A modern C++ library for type-safe environment variable parsing - ph3at/libenvpp
- 287Spack
https://spack.io/
A flexible package manager supporting multiple versions, configurations, platforms, and compilers.
- 288C++ Build Benchmarks
https://build-bench.com/
Compare build times of C++ code
- 289Simple Dynamic Strings library for C
https://github.com/antirez/sds
Simple Dynamic Strings library for C. Contribute to antirez/sds development by creating an account on GitHub.
- 290A Discord API wrapper library made in C
https://github.com/Cogmasters/concord
A Discord API wrapper library made in C. Contribute to Cogmasters/concord development by creating an account on GitHub.
- 291C++ Web Framework REST API
https://github.com/wfrest/wfrest
C++ Web Framework REST API. Contribute to wfrest/wfrest development by creating an account on GitHub.
- 292High performance C++11 signals
https://github.com/larspensjo/SimpleSignal
High performance C++11 signals. Contribute to larspensjo/SimpleSignal development by creating an account on GitHub.
- 293Implementation of python itertools and builtin iteration functions for C++17
https://github.com/ryanhaining/cppitertools
Implementation of python itertools and builtin iteration functions for C++17 - ryanhaining/cppitertools
- 294static analysis of C/C++ code
https://github.com/danmar/cppcheck
static analysis of C/C++ code. Contribute to danmar/cppcheck development by creating an account on GitHub.
- 295Anjuta DevStudio
https://sourceforge.net/projects/anjuta/
Download Anjuta DevStudio for free. Anjuta DevStudio is a versatile Integrated Development Environment (IDE) for software development on GNU/Linux. It features many advanced facilities such as project management, application wizards, interactive debugger, source browsing etc.
- 296Online C++ Compiler - Programiz
https://www.programiz.com/cpp-programming/online-compiler
Write and run your C++ code using our online compiler. Enjoy additional features like code sharing, dark mode, and support for multiple programming languages.
- 297libonion - Coralbits S.L.
http://www.coralbits.com/libonion/
Lightweight C library to add web server functionality to your program libonion is a lightweight library to help you create webservers in C programming language. These webservers may be a web application, a means of expanding your own application to give it web functionality or even a fully featured webserver. The user can create new […]
- 298Learn C++ - Best C++ Tutorials | Hackr.io
https://hackr.io/tutorials/learn-c-plus-plus
Learning C++? Check out these best online C++ courses and tutorials recommended by the programming community. Pick the tutorial as per your learning style: video tutorials or a book. Free course or paid. Tutorials for beginners or advanced learners. Check C++ community's reviews & comments.
- 299C++ Team Blog
https://devblogs.microsoft.com/cppblog/
C++ tutorials, C and C++ news, and information about Visual Studio, Visual Studio Code, and Vcpkg from the Microsoft C++ team.
- 300Rapid YAML - a library to parse and emit YAML, and do it fast.
https://github.com/biojppm/rapidyaml
Rapid YAML - a library to parse and emit YAML, and do it fast. - biojppm/rapidyaml
- 301CppExpert | The best way to Learn C++ for free
https://cppexpert.online
CppExpert is the best free platform to learn C++. Learn the best practices of C++ programming with CppExpert.
- 302Smart Swift/Objective-C IDE for iOS & macOS Development
http://www.jetbrains.com/objc/
An intelligent IDE for iOS/macOS development focused on code quality, efficient code navigation, smart code completion, on-the-fly code analysis with quick-fixes and superior code refactorings.
- 303A Cross-Platform IDE for C and C++ by JetBrains
http://www.jetbrains.com/clion/
A powerful IDE from JetBrains helps you develop in C and C++ on Linux, macOS and Windows.
- 304.NET programming with C++/CLI
https://docs.microsoft.com/en-us/cpp/dotnet/dotnet-programming-with-cpp-cli-visual-cpp?view=msvc-160
Learn how to use C++/CLI to create .NET apps and components in Visual Studio.
- 305Home · Wiki · GNOME / libxml2 · GitLab
http://xmlsoft.org/
XML parser and toolkit
- 306C++ quiz
https://quiz.pvs-studio.com
Test yourself. See if you can find all the bugs in the code.
- 307C++11/14/17/20 library for lazy evaluation
https://github.com/MarcDirven/cpp-lazy
C++11/14/17/20 library for lazy evaluation. Contribute to MarcDirven/cpp-lazy development by creating an account on GitHub.
- 308Ideone.com
http://ideone.com/
Ideone is something more than a pastebin; it's an online compiler and debugging tool which allows to compile and run code online in more than 40 programming languages.
- 309GotW
https://herbsutter.com/gotw/
Guru of the Week (GotW) is a series of C++ programming problems created and written by Herb Sutter. Starting in May 2013, GotW articles are currently being revised to match the upcoming C++14 ISO S…
- 310Dev-C++
http://sourceforge.net/projects/orwelldevcpp/
Download Dev-C++ for free. A free, portable, fast and simple C/C++ IDE. A new and improved fork of Bloodshed Dev-C++
- 311Mastering MISRA C++ Compliance with CppDepend.
https://www.cppdepend.com/misra-cpp
Discover the role of MISRA C++ in the automotive industry, a collaborative effort between vehicle manufacturers, component suppliers, and engineering consultancies to establish best practices for safety-related electronic systems in road vehicles and other embedded systems. Learn how CppDepend supports MISRA C++ compliance to achieve safer and more reliable software.
- 312Online Courses - Learn Anything, On Your Schedule | Udemy
https://www.udemy.com/topic/C-plus-plus-tutorials/
Udemy is an online learning and teaching marketplace with over 250,000 courses and 73 million students. Learn programming, marketing, data science and more.
- 313boostcon/cppnow_presentations_2022
https://github.com/boostcon/cppnow_presentations_2022
Contribute to boostcon/cppnow_presentations_2022 development by creating an account on GitHub.
- 314IDE and Code Editor for Software Developers and Teams
https://www.visualstudio.com/
Visual Studio dev tools & services make app development easy for any developer, on any platform & language. Develop with our code editor or IDE anywhere for free.
- 315C++ Tutorial | Learn C++ Programming Language - Scaler Topics
https://www.scaler.com/topics/cpp
This C++ tutorial on Scaler Topics will teach you all concepts in C++, from the fundamentals to the advanced concepts. Beginners and even professionals can easily follow this C++ tutorial.
- 316Repository for the slides and the code of my "Quick game development with C++11/C++14" CppCon 2014 talk.
https://github.com/SuperV1234/cppcon2014
Repository for the slides and the code of my "Quick game development with C++11/C++14" CppCon 2014 talk. - vittorioromeo/cppcon2014
- 317C/C++ language server supporting multi-million line code base, powered by libclang. Emacs, Vim, VSCode, and others with language server protocol support. Cross references, completion, diagnostics, semantic highlighting and more
https://github.com/cquery-project/cquery/
C/C++ language server supporting multi-million line code base, powered by libclang. Emacs, Vim, VSCode, and others with language server protocol support. Cross references, completion, diagnostics, ...
- 318Intel® oneAPI DPC++/C++ Compiler
https://software.intel.com/en-us/c-compilers
Compile for CPUs, GPUs, and FPGAs with an LLVM technology-based compiler that enables custom accelerator tuning and supports OpenMP for GPU offload.
- 319Replit – Build software faster
https://repl.it
Replit is an AI-powered software development & deployment platform for building, sharing, and shipping software fast.
- 320Presentation on Hana for C++Now 2015
https://github.com/ldionne/hana-cppnow-2015
Presentation on Hana for C++Now 2015. Contribute to ldionne/cppnow-2015-hana development by creating an account on GitHub.
- 321Eclipse CDT™ (C/C++ Development Tooling)
http://www.eclipse.org/cdt/
Eclipse CDT™ (C/C++ Development Tooling) has 7 repositories available. Follow their code on GitHub.
- 322Collaborative Collection of C++ Best Practices. This online resource is part of Jason Turner's collection of C++ Best Practices resources. See README.md for more information.
https://github.com/lefticus/cppbestpractices
Collaborative Collection of C++ Best Practices. This online resource is part of Jason Turner's collection of C++ Best Practices resources. See README.md for more information. - cpp-best-practic...
- 323ruslo/hunter
https://www.github.com/ruslo/hunter
Contribute to ruslo/hunter development by creating an account on GitHub.
- 324This project is obsolete. TinyXML-2 offers a very similar C++ interface.
https://github.com/rjpcomputing/ticpp
This project is obsolete. TinyXML-2 offers a very similar C++ interface. - wxFormBuilder/ticpp
- 325Suite of C++ libraries for radio astronomy data processing
https://code.google.com/p/casacore/
Suite of C++ libraries for radio astronomy data processing - casacore/casacore
- 326A C++ implementation of timsort
https://github.com/gfx/cpp-TimSort
A C++ implementation of timsort. Contribute to timsort/cpp-TimSort development by creating an account on GitHub.
- 327Drogon: A C++14/17/20 based HTTP web application framework running on Linux/macOS/Unix/Windows
https://github.com/an-tao/drogon
Drogon: A C++14/17/20 based HTTP web application framework running on Linux/macOS/Unix/Windows - drogonframework/drogon
- 328Cross-platform C++11 header-only library for memory mapped file IO
https://github.com/mandreyel/mio
Cross-platform C++11 header-only library for memory mapped file IO - vimpunk/mio
Related Articlesto learn about angular.
- 1Introduction to C++: Beginner's Guide to Getting Started
- 2C++ Variables, Data Types, and Operators
- 3Control Flow in C++: Conditionals and Loops
- 4C++ Object-Oriented Programming: Classes and Objects
- 5Templates and Generic Programming in C++: Comprehensive Guide
- 6C++ Memory Management: Pointers and Dynamic Allocation
- 7Building Your First C++ Application: Step-by-Step Guide
- 8C++ in Game Development: How to Get Started with Simple Games
- 9Best Practices for Writing Efficient and Maintainable C++ Code
- 10Advanced C++ Optimization: Performance in Critical Areas
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here 🔍.
- 1
how long does it take to learn c++ programming
- 2
what c++ programming language
- 3
where to practice c++ programming
- 4
should i learn c++ or python
- 5
why was c++ invented
- 6
what are variables in c++ programming
- 7
which app is used for c++ programming
- 8
how to do c programming in turbo c++
- 9
what does mean in c++ programming
- 10
where c++ programming is used
- 11
how to learn c++ programming for beginners
- 13
is c++ programming language still used
- 14
why c++ is best for competitive programming
- 15
why was c++ developed
- 16
when is c++ used more often
- 17
what is c++ programming language definition
- 18
how to use dev c++ for c programming
- 19
why c++ procedural programming
- 20
what are strings in c++ programming
- 21
what is generic programming in c++
- 22
who developed c++ programming language
- 23
what does a c++ programmer do
- 24
where to learn c++ programming
- 25
where to learn c++ for competitive programming
- 26
when is c++ used
- 27
how to learn c++ programming language
- 28
why we use c++ programming language
- 29
who created c++ programming language
- 30
what can you do with c++ programming
- 31
how to do c++ programming in ubuntu
- 32
what are advantages of java programming over c++
- 33
what programming language is c++ written in
- 34
what c++ programming is used for
- 35
should i learn c++ or java first
- 36
how to practice c++ programming
- 37
how to use turbo c++ for c programming
- 38
should i learn c++ or python first
- 39
which app is best for c++ programming
- 40
how to learn c++ programming
- 41
can c++ run on mac
- 42
when was c++ programming language invented
- 43
does c++ support functional programming
- 44
is c++ a programming language
- 45
should i learn c++ as a beginner
- 46
what can i do with c++ programming language
- 47
what is an array in programming c++
- 48
why does c++ fail in web programming
- 49
can c and c++ be mixed
- 50
will c++ become obsolete
- 51
does c++ have a future
- 52
can c++ run on linux
- 53
how to do c++ programming in android phone
- 54
what are the function in c++ programming
- 55
should i learn c++ as my first language
- 56
where to download c++ programming language
- 57
what are the features of object oriented programming in c++
- 58
was c++ written in c
- 59
how to start c++ programming language
- 60
how can i learn c++ programming language
- 61
why c++ is the best programming language
- 62
what are the uses of c++ programming language
- 63
why is c++ used in competitive programming
- 64
can c++ call python
- 65
how to use c++ programming language
- 66
will c++ ever die
- 67
does c++ cost money
- 68
when c++ is mostly used
- 69
is c++ programming hard
- 70
who invented c++ programming language
- 71
which software is used for c++ programming
- 72
where to do c++ programming
- 73
how do i learn c++ programming language
- 74
how to make a programming language in c++
- 75
can c++ run c code
- 76
why is c++ called object oriented programming
- 77
how do you pronounce c++ programming language
- 78
what is c++ programming language in hindi
- 79
why is c++ object oriented programming
- 80
who updates c++
- 81
what is c++ programming with example
- 82
what are the characteristics of c++ programming language
- 83
what is introduction to c++ programming
- 84
what can you do with c++ programming language
- 85
why learn c++ programming
- 86
which programming language should i learn after c++
- 87
who uses c++ programming language
- 88
how to do c++ programming in windows 10
- 89
what is c++ programming language used for
- 90
what are the data types in c++ programming
- 91
how did c++ get its name
- 92
what is c++ in computer programming
- 93
who created c++
- 94
what is procedure oriented programming in c++
- 95
how to start learning c++ programming
- 96
what is c++ programming used for
- 97
is c++ programming language
- 98
is c++ good for software development
- 99
is c++ powerful
- 100
what is basic c++ program
- 101
what is c++ in coding
- 102
what is c++ programming pdf
- 103
which software is best for c++ programming
- 104
what is c++ programming
- 105
why is c++ important in programming
- 106
what are structures in c++ programming
- 107
when did c++ come out
- 108
what is object oriented programming in c++
- 109
when was c++ invented
- 110
when was c++ programming language created
- 111
why study c++ programming
- 112
will c++ be replaced
- 113
does c code work in c++
More Sitesto check out once you're finished browsing here.
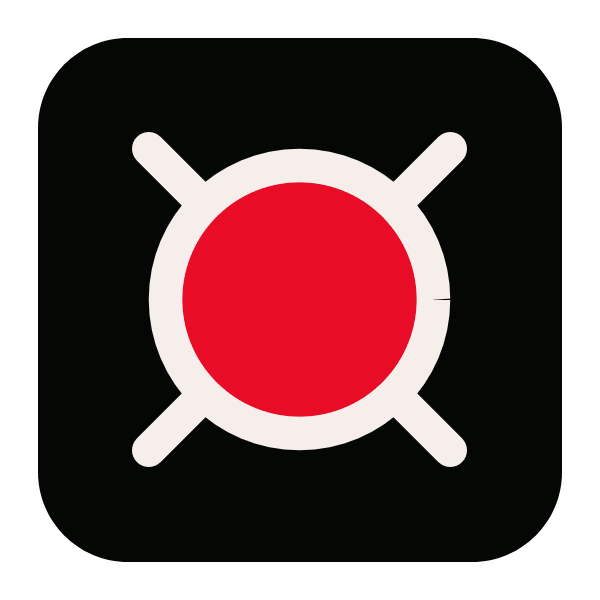
https://www.0x3d.site/
0x3d is designed for aggregating information.
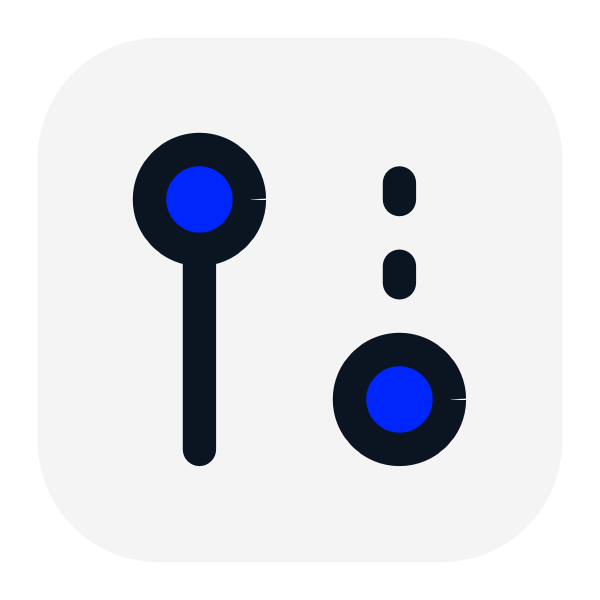
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
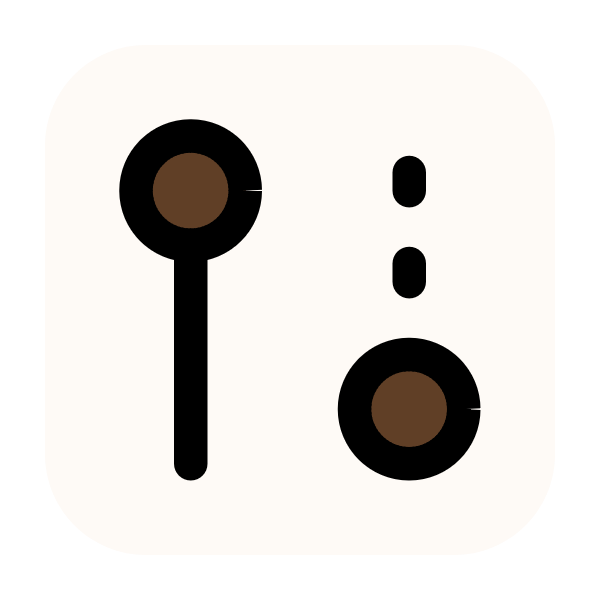
https://open-source.0x3d.site/
Open Source Online Directory
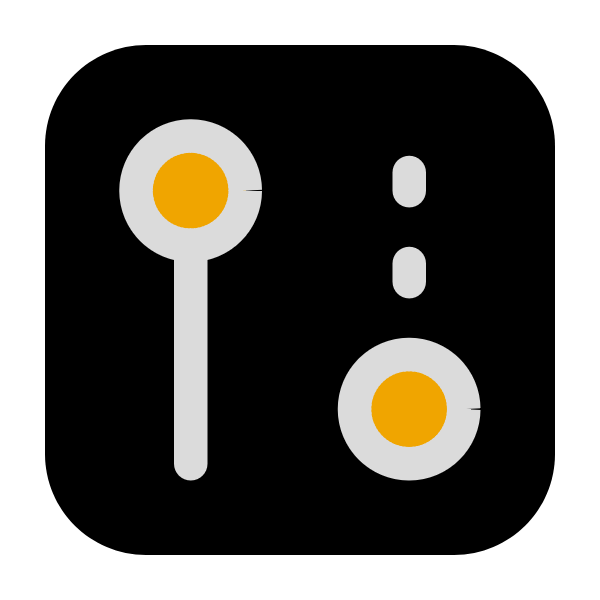
https://analytics.0x3d.site/
Analytics Online Directory
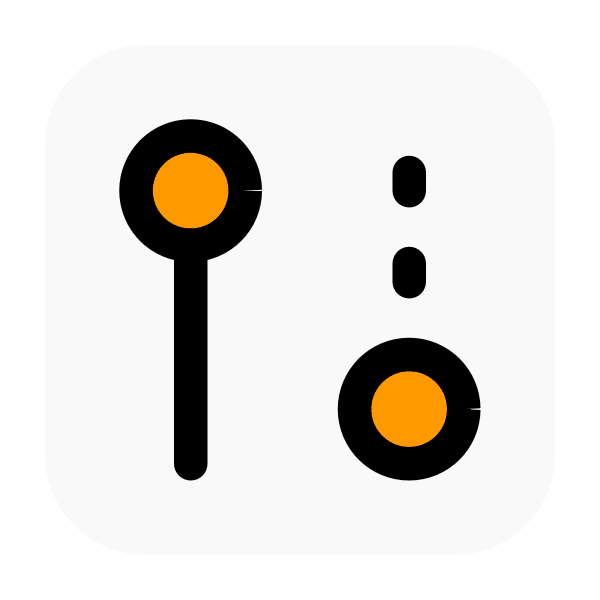
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
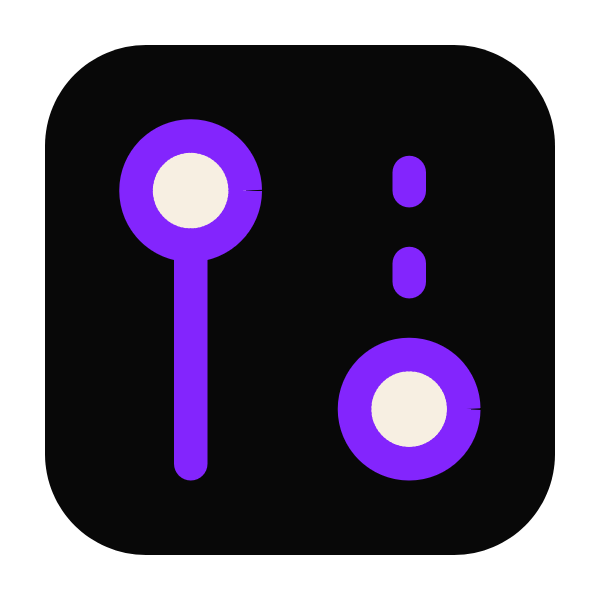
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
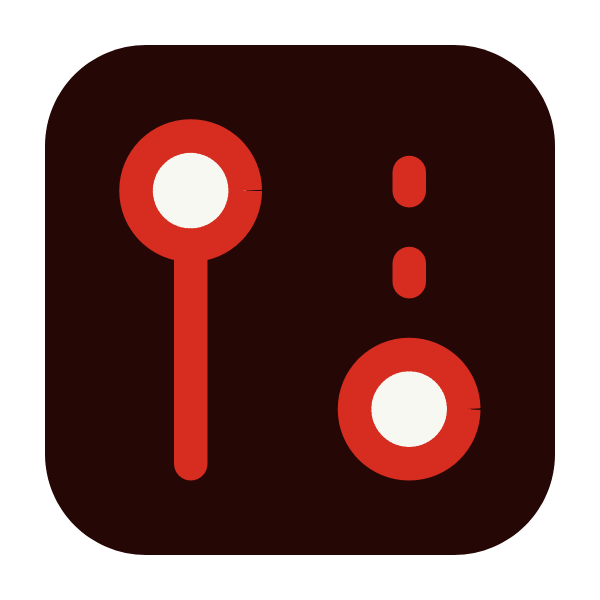
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
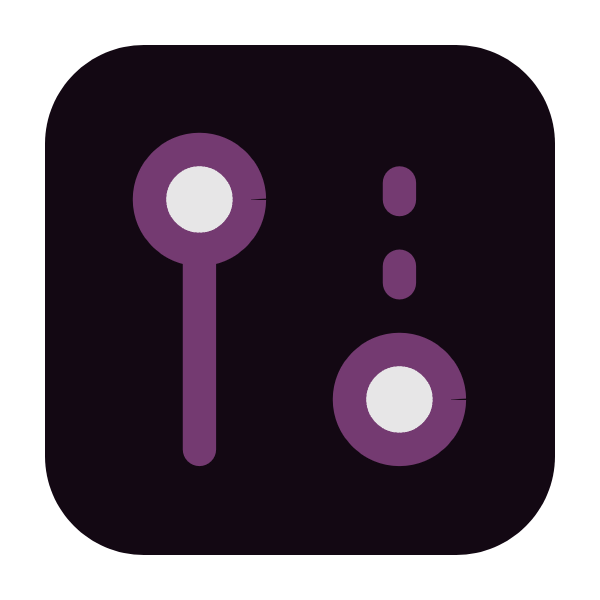
https://elixir.0x3d.site/
Elixir Online Directory
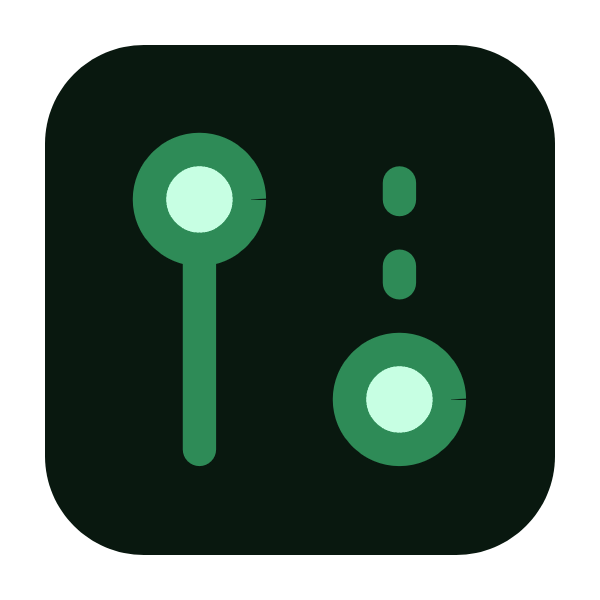
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
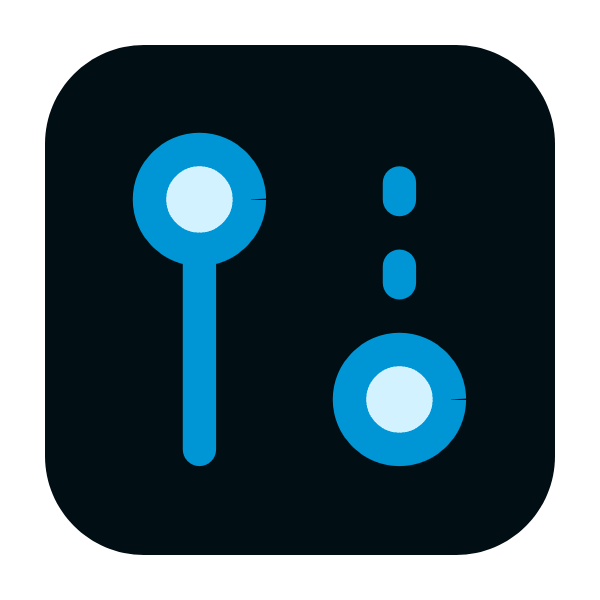
https://c-programming.0x3d.site/
C Programming Online Directory
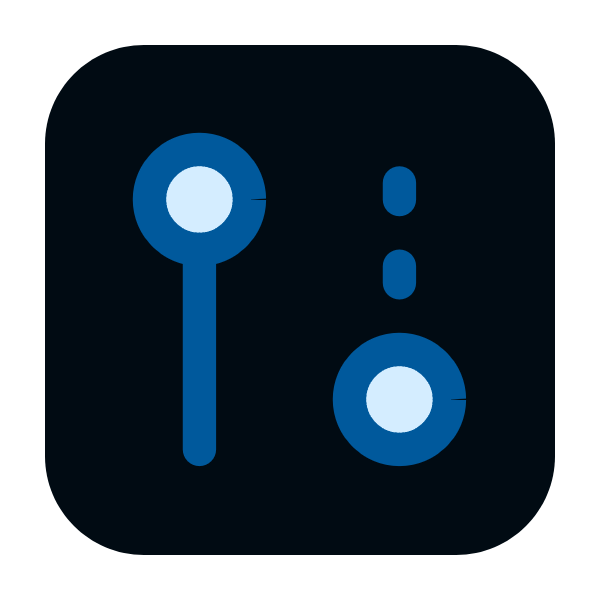
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
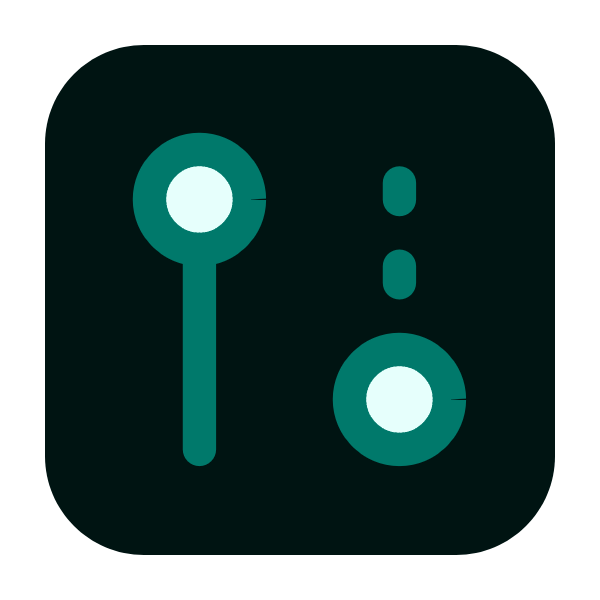
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
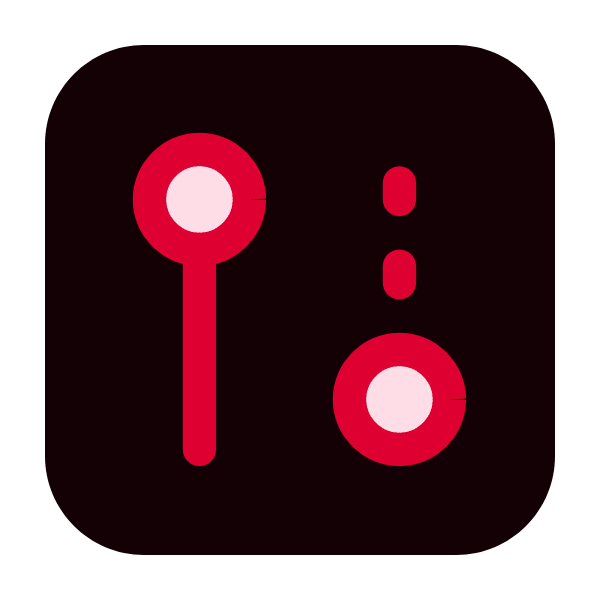
https://angular.0x3d.site/
Angular JS Online Directory